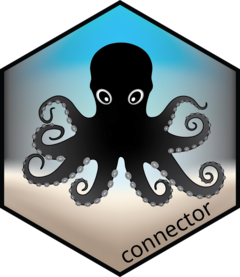
Use dplyr verbs to interact with the remote database table
Source:R/cnt_generics.R
, R/dbi_methods.R
, R/fs_methods.R
, and 1 more
tbl_cnt.Rd
Generic implementing of how to create a dplyr::tbl()
connection in order
to use dplyr verbs to interact with the remote database table.
Mostly relevant for DBI connectors.
ConnectorDBI: Uses
dplyr::tbl()
to create a table reference to a table in a DBI connection.
ConnectorFS: Uses
read_cnt()
to allow redundancy between fs and dbi.
Usage
tbl_cnt(connector_object, name, ...)
# S3 method for class 'ConnectorDBI'
tbl_cnt(connector_object, name, ...)
# S3 method for class 'ConnectorFS'
tbl_cnt(connector_object, name, ...)
# S3 method for class 'ConnectorLogger'
tbl_cnt(connector_object, name, ...)
Value
A dplyr::tbl object.
Examples
# Use dplyr verbs on a table in a DBI database
cnt <- connector_dbi(RSQLite::SQLite())
iris_cnt <- cnt |>
write_cnt(iris, "iris") |>
tbl_cnt("iris")
iris_cnt
#> # Source: table<`iris`> [?? x 5]
#> # Database: sqlite 3.50.1 []
#> Sepal.Length Sepal.Width Petal.Length Petal.Width Species
#> <dbl> <dbl> <dbl> <dbl> <chr>
#> 1 5.1 3.5 1.4 0.2 setosa
#> 2 4.9 3 1.4 0.2 setosa
#> 3 4.7 3.2 1.3 0.2 setosa
#> 4 4.6 3.1 1.5 0.2 setosa
#> 5 5 3.6 1.4 0.2 setosa
#> 6 5.4 3.9 1.7 0.4 setosa
#> 7 4.6 3.4 1.4 0.3 setosa
#> 8 5 3.4 1.5 0.2 setosa
#> 9 4.4 2.9 1.4 0.2 setosa
#> 10 4.9 3.1 1.5 0.1 setosa
#> # ℹ more rows
iris_cnt |>
dplyr::collect()
#> # A tibble: 150 × 5
#> Sepal.Length Sepal.Width Petal.Length Petal.Width Species
#> <dbl> <dbl> <dbl> <dbl> <chr>
#> 1 5.1 3.5 1.4 0.2 setosa
#> 2 4.9 3 1.4 0.2 setosa
#> 3 4.7 3.2 1.3 0.2 setosa
#> 4 4.6 3.1 1.5 0.2 setosa
#> 5 5 3.6 1.4 0.2 setosa
#> 6 5.4 3.9 1.7 0.4 setosa
#> 7 4.6 3.4 1.4 0.3 setosa
#> 8 5 3.4 1.5 0.2 setosa
#> 9 4.4 2.9 1.4 0.2 setosa
#> 10 4.9 3.1 1.5 0.1 setosa
#> # ℹ 140 more rows
iris_cnt |>
dplyr::group_by(Species) |>
dplyr::summarise(
n = dplyr::n(),
mean.Sepal.Length = mean(Sepal.Length, na.rm = TRUE)
) |>
dplyr::collect()
#> # A tibble: 3 × 3
#> Species n mean.Sepal.Length
#> <chr> <int> <dbl>
#> 1 setosa 50 5.01
#> 2 versicolor 50 5.94
#> 3 virginica 50 6.59
# Use dplyr verbs on a table
folder <- withr::local_tempdir()
cnt <- connector_fs(folder)
cnt |>
write_cnt(iris, "iris.csv")
#> Error: Cannot open file for writing:
#> * '/tmp/RtmpnpFke0/file210679c2faed/iris.csv'
iris_cnt <- cnt |>
tbl_cnt("iris.csv")
#> Error in find_file(name, root = connector_object$path): No file found or multiple files found with the same name
iris_cnt
#> # Source: table<`iris`> [?? x 5]
#> # Database: sqlite 3.50.1 []
#> Sepal.Length Sepal.Width Petal.Length Petal.Width Species
#> <dbl> <dbl> <dbl> <dbl> <chr>
#> 1 5.1 3.5 1.4 0.2 setosa
#> 2 4.9 3 1.4 0.2 setosa
#> 3 4.7 3.2 1.3 0.2 setosa
#> 4 4.6 3.1 1.5 0.2 setosa
#> 5 5 3.6 1.4 0.2 setosa
#> 6 5.4 3.9 1.7 0.4 setosa
#> 7 4.6 3.4 1.4 0.3 setosa
#> 8 5 3.4 1.5 0.2 setosa
#> 9 4.4 2.9 1.4 0.2 setosa
#> 10 4.9 3.1 1.5 0.1 setosa
#> # ℹ more rows
iris_cnt |>
dplyr::group_by(Species) |>
dplyr::summarise(
n = dplyr::n(),
mean.Sepal.Length = mean(Sepal.Length, na.rm = TRUE)
)
#> # Source: SQL [?? x 3]
#> # Database: sqlite 3.50.1 []
#> Species n mean.Sepal.Length
#> <chr> <int> <dbl>
#> 1 setosa 50 5.01
#> 2 versicolor 50 5.94
#> 3 virginica 50 6.59